[TOC] #### 1. JS 正則表達(dá)式 --- JS 正則表達(dá)式語法: ``` # JS 的正則表達(dá)式不需要使用引號包裹,PHP 需要使用引號包裹。修飾符是可選的,可寫可不寫 /正則表達(dá)式主體/修飾符 ``` JS 中使用正則表達(dá)式的方法比較多,可以按照使用兩種類型記憶: 字符串對象方法、正則表達(dá)式對象方法 ```javascript // 字符串對象方法 string.search(regexp) // 正則表達(dá)式對象方法 regexp.test(string) ``` #### 2. 使用字符串方法 --- `string.search(regexp)` 匹配首次出現(xiàn)的下標(biāo) ```javascript const string = 'hello world !' // 返回內(nèi)容首次出現(xiàn)的位置(下標(biāo)),沒有匹配到時返回 -1 const index = string.search(/world/) ``` `string.replace(regexp, new_string)` 將首次匹配到的內(nèi)容進(jìn)行替換 ```javascript const string = 'hello world !' // 將首次匹配到的內(nèi)容進(jìn)行替換 const result = string.replace(/world/, 'vue') ``` `string.match(regexp)` 執(zhí)行正則表達(dá)式匹配 ```javascript const string = 'hello world !' // 下面 result1 和 result2 結(jié)果相同 // ['world', index: 6, input: 'hello world !', groups: undefined] const result1 = string.match(/world/) const result2 = /world/.exec(string) ``` `string.matchAll(regexp)` 執(zhí)行正則表達(dá)式匹配,匹配字符串所有符合條件的內(nèi)容 ```javascript const string = 'hello world world !' const result = [...string.matchAll(/world/g)] console.log(result); ``` 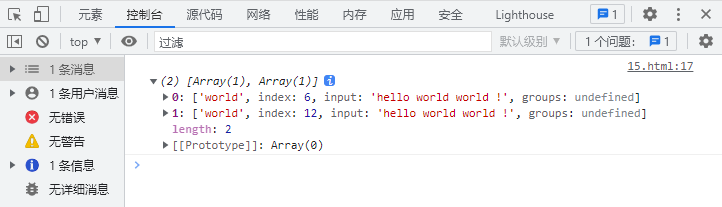 #### 3. 使用 RegExp 方法 --- `regexp.test(string)` 用于檢測一個字符串是否匹配某個模式 ```javascript const string = 'hello world !' const bool = /world/.test(string) ``` `regexp.exec(string)` 執(zhí)行正則表達(dá)式匹配,匹配成功時返回一個數(shù)組,匹配失敗返回 null ```javascript const string = 'hello world !' // ['world', index: 6, input: 'hello world !', groups: undefined] const result = /world/.exec(string) ```